- Amir Boroumand | Software Engineer based in Pittsburgh, PA/
- blog/
- Create List and Map Beans in Spring XML/
Create List and Map Beans in Spring XML
·1 min
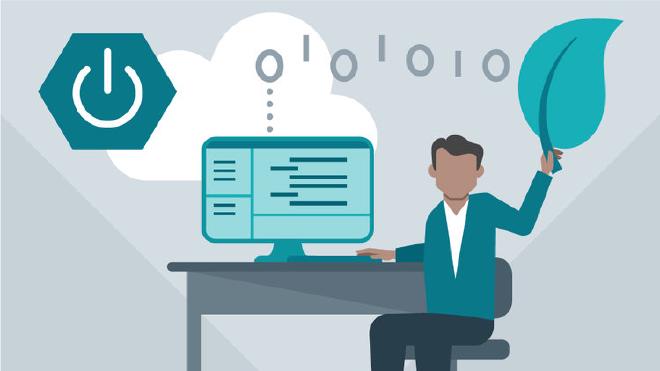
Overview #
In this article, we’ll explore how to create lists and maps in the Spring Framework using XML configuration.
Util Namespace #
First, we need to make sure that the Spring util namespace is imported at the top of the file.
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:util="http://www.springframework.org/schema/util"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/util
http://www.springframework.org/schema/util/spring-util.xsd">
</beans>
Creating Lists #
We can define a list bean as follows:
<util:list id="namesList" value-type="java.lang.String">
<value>Amir</value>
<value>Arnie</value>
<value>Beth</value>
<value>Lucy</value>
</util:list>
The value-type
attribute is optional but useful to include since it makes it clear what types of objects the list contains.
The default list implementation is ArrayList
. To use a different implementation, include the list-class attribute:
<util:list id="namesList" list-class="java.util.LinkedList" value-type="java.lang.String">
<value>Amir</value>
<value>Arnie</value>
<value>Beth</value>
<value>Lucy</value>
</util:list>
We can reference other beans in our list as well:
<bean id="maxLengthValidator" class="com.codebyamir.data.Validator" />
<bean id="formatValidator" class="com.codebyamir.data.Validator" />
<util:list id="validators" value-type="com.codebyamir.data.Validator">
<ref bean="maxLengthValidator"/>
<ref bean="formatValidator"/>
</util:list>
Creating Maps #
Similarly, we can define a map as follows:
<util:map id="responsiveImageFormats" map-class="java.util.HashMap" key-type="java.lang.String" value-type="java.lang.Integer">
<entry key="widescreen" value="1200"/>
<entry key="desktop" value="992"/>
<entry key="tablet" value="768"/>
<entry key="mobile" value="480"/>
</util:map>