- Amir Boroumand | Software Engineer based in Pittsburgh, PA/
- blog/
- Do You Still Need Apache Commons StringUtils in Modern Java?/
Do You Still Need Apache Commons StringUtils in Modern Java?
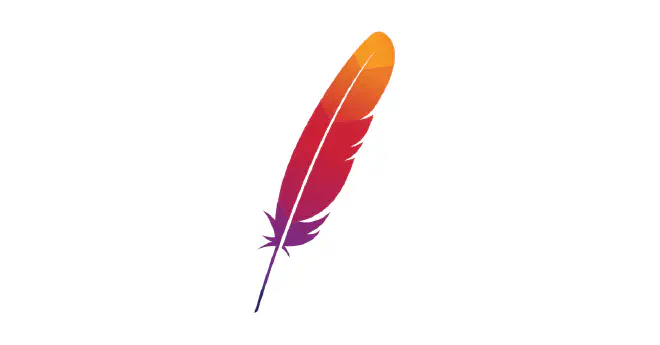
Introduction #
For years, Java developers relied on Apache Commons Lang’s StringUtils
to handle common string operations that the Java standard library lacked. From null-safe string checks to string joins and abbreviations, StringUtils
provided convenient static methods that simplified Java string manipulation.
With the release of Java 8 and later versions (especially Java 11 and Java 17), the standard library has native methods that perform the same operations.
Null-Safe String Checks #
One of the most commonly used features of StringUtils
was checking for null or empty strings without triggering a NullPointerException
.
Apache Commons StringUtils
if (StringUtils.isEmpty(name)) { } // Returns true for null or ""
if (StringUtils.isNotEmpty(name)) { }
if (StringUtils.isBlank(name)) { } // Also checks for whitespace-only strings
if (StringUtils.isNotBlank(name)) { }
Modern Java Alternative (Java 11+)
if (name == null || name.isEmpty()) { } // Equivalent to isEmpty()
if (name != null && !name.isEmpty()) { } // Equivalent to isNotEmpty()
if (name == null || name.isBlank()) { } // Equivalent to isBlank()
if (name != null && !name.isBlank()) { } // Equivalent to isNotBlank()
The Apache Commons methods are more readable. But Java 11 did introduce String.isBlank()
.
String Joining #
Prior to Java 8, joining strings with a separator was verbose, making StringUtils.join()
popular.
Apache Commons StringUtils
List<String> names = Arrays.asList("Arnie", "Lucy", "Beth", "Amir");
String joined = StringUtils.join(names, ","); // "Arnie,Lucy,Beth,Amir"
Modern Java Alternative (Java 8+)
String joined = String.join(",", names);
Here I prefer the Java alternative especially since it natively supports lists and arrays.
String Truncation #
The StringUtils.abbreviate()
method truncates a string and appends an ellipsis (…).
Apache Commons StringUtils
String sentence = "The cat lept over the big brown dog.";
String abbreviated = StringUtils.abbreviate(sentence, 20); // "The cat lept ov..."
Modern Java Alternative (Java 8+)
String sentence = "The cat lept over the big brown dog.";
String ellipsis = "...";
String abbreviated = sentence.length() > 17 ? sentence.substring(0, 14) + ellipsis : sentence;
Numeric String Checks #
Checking if a string contains only digits was a common use case for StringUtils.
Apache Commons StringUtils
if (StringUtils.isNumeric(str)) { } // True if str contains only digits
Modern Java Alternative (Java 8+)
if (str != null && str.matches("\\d+")) { }
Or using Java Streams:
boolean isNumeric = str.chars().allMatch(Character::isDigit);
Case Conversion #
Converting the first letter of a string to uppercase or lowercase was a common use case.
Apache Commons StringUtils
String capitalized = StringUtils.capitalize("hello"); // "Hello"
String uncapitalized = StringUtils.uncapitalize("Hello"); // "hello"
Modern Java Alternative (Java 8+)
String capitalized = str.substring(0, 1).toUpperCase() + str.substring(1);
String uncapitalized = str.substring(0, 1).toLowerCase() + str.substring(1);
String Reversal #
Reversing a string is another common need.
Apache Commons StringUtils
String reversed = StringUtils.reverse("hello"); // "olleh"
Modern Java Alternative (Java 8+)
String reversed = new StringBuilder("hello").reverse().toString();
Is StringUtils Still Useful? #
With Java 8, 11, and 17, many of the features provided by StringUtils
are available in the native language. However, there are still cases where Apache Commons Lang is useful:
- When working with legacy Java versions (< Java 8)
- Better code readability
- Handling null checks and edge cases
- For additional string utilities (e.g., StringUtils.leftPad(), difference())
- If already used in your codebase (consistency matters!)
However, if you’re starting a new project on Java 11+, consider using built-in Java methods instead of Apache Commons Lang.