- Amir Boroumand | Software Engineer based in Pittsburgh, PA/
- blog/
- When to Use an Abstract Class in Java/
When to Use an Abstract Class in Java
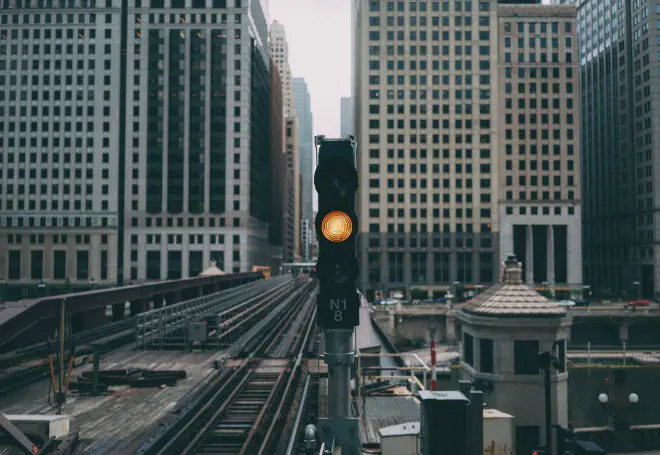
Overview #
One of the fundamental concepts in OOP is the abstract class. Abstract classes cannot be instantiated and are designed to be subclassed. They are used to provide some common functionality across a set of related classes while also allowing default method implementations.
In this article, we’ll discuss a real world scenario for using an abstract class in Java.
Example Application #
Let’s say we are developing an application that accepts several types of orders: store orders, warehouse orders, and online orders.
Our requirements state the following:
- All orders share some common properties like order ID, a list of products, date/time created, and amount
- Some types of orders may contain their own specific properties (store ID, store representative, etc)
- Order validation and processing logic are different based on type
- Order cancellation logic is universal across all types
Implementations #
Solution 1 - A Single Concrete Class #
If we simply created a concrete class for Order, it would quickly become convoluted for a couple reasons:
- Some of our class fields don’t make sense for certain orders (an online order doesn’t need a store ID or store rep)
- The
validate()
andprocess()
methods need to check the order type before executing any business logic.
Order.java [concrete] #
package com.steelcityamir.demo;
import java.math.BigDecimal;
import java.time.LocalDate;
public class Order {
public enum OrderType {
ONLINE, STORE, WAREHOUSE;
}
private long id;
private long storeId;
private Employee storeRep;
private long warehouseId;
private LocalDate createdOn;
private BigDecimal amount;
private OrderType type;
private List<Product> products;
public void validate() {
if (type == OrderType.STORE) {
// code for store order validation
} else if (type == OrderType.WAREHOUSE) {
// code for warehouse order validation
} else if (type == OrderType.ONLINE) {
// code for online order validation
}
}
public void process() {
if (type == OrderType.STORE) {
// code for store order validation
} else if (type == OrderType.WAREHOUSE) {
// code for warehouse order validation
} else if (type == OrderType.ONLINE) {
// code for online order validation
}
}
public void cancel() {
// universal code for order cancellations
}
}
Moreover, if the business introduced a new order type, we would need to modify this class. This is a violation of the Open/Closed principle of SOLID.
Solution 2 - Single Base Abstract class with Subclasses for Each Type #
A cleaner solution is to implement a base abstract class called Order and subclass the different types.
When it’s time to add a new order type, we don’t need to touch the Order class at all. We would simply create a new subclass that extends Order.
Order.java [abstract] #
Here we add the abstract
keyword to the class and the validate()
and process()
methods. We also make our cancel()
method final so it cannot be overridden by its subclasses.
package com.steelcityamir.demo;
import java.math.BigDecimal;
import java.time.LocalDate;
public abstract class Order {
private long id;
private LocalDate createdOn;
private BigDecimal amount;
public abstract void validate();
public abstract void process();
public final void cancel() {
// universal code for order cancellations
}
}
StoreOrder.java [subclass] #
Our subclass for store orders contains fields for store ID, store rep, and custom implementations for validation and processing.
package com.steelcityamir.demo;
public class StoreOrder extends Order {
private long storeId;
private Employee storeRep;
@Override
public void validate() {
// TODO Auto-generated method stub
}
@Override
public void process() {
// TODO Auto-generated method stub
}
}
WarehouseOrder.java [subclass] #
Our subclass for warehouse orders contains fields for warehouse ID and custom implementations for validation and processing.
package com.steelcityamir.demo;
public class WarehouseOrder extends Order {
private long warehouseId;
@Override
public void validate() {
// TODO Auto-generated method stub
}
@Override
public void process() {
// TODO Auto-generated method stub
}
}
OnlineOrder.java [subclass] #
Our subclass for online orders contain fields for coupon code and custom implementations for validation and processing.
package com.steelcityamir.demo;
public class OnlineOrder extends Order {
private String couponCode;
@Override
public void validate() {
// TODO Auto-generated method stub
}
@Override
public void process() {
// TODO Auto-generated method stub
}
}
Rules to Remember #
- Abstract classes cannot be instantiated.
- If a class has at least one abstract method, then the class must be declared abstract.
- To use an abstract class, we must create a class that extends the abstract class (inheritance) and provide implementations for all abstract methods.
- Java does not support multiple inheritance so we are only allowed to extend one class (abstract or not).There is where interfaces become useful.